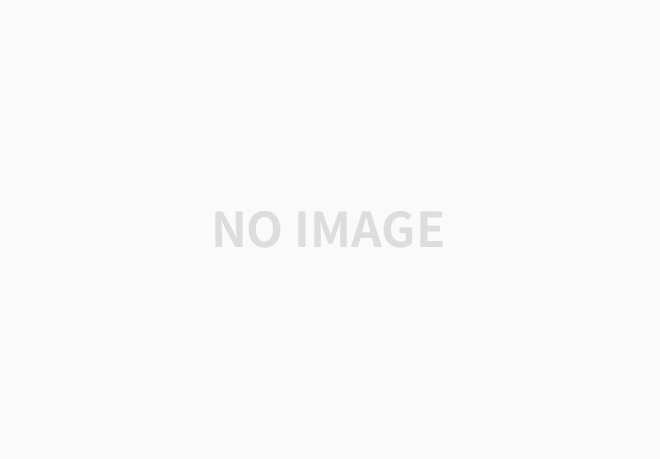
클래스 상속 선언법
class 자식클래스 extends 부모클래스{
// 필드
// 생성자
// 메소드
}
부모생성자 호출 super()
자식클래스(매개변수선언, ...){
super(매개값, ...);
}
메소드 재정의
자식클래스에서 부모 클래스의 메소드를 다시 정의하는 것으로
- 부모 메소드와 동일한 리턴 타입, 메소드 이름, 매개 변수 목록을 가져야 한다.
- 접근 제한을 더 강하게 재정의 할 수 없다.
- 새로운 예외를 throws 할 수 없다.
부모 메소드 호출
super.부모메소드();
상속할 수 없는 final 클래스
클래스를 선언할 때 final 키워드를 class 앞에 붙이면 이 클래스는 최종적인 클래스이므로 상속할 수 없는 클래스가 된다. (부모 클래스가 될 수 없어 자식 클래스를 만들 수 없다.)
public final class 클래스 { ... }
재정의할 수 없는 final 메소드
메소드를 선언할 때 final을 붙이면 최종적인 메소드이므로 재정의할 수 없는 메소드가 된다. (부모 클래스를 상속해서 자식 클래스를 선언할 때 부모 클래스에 선언된 final 메소드는 자식 클래스에서 재정의 할 수 없다.)
public final 리턴타입 메소드( [ 메개변수, ...] ) { ... }
+ 상속 활용해보기
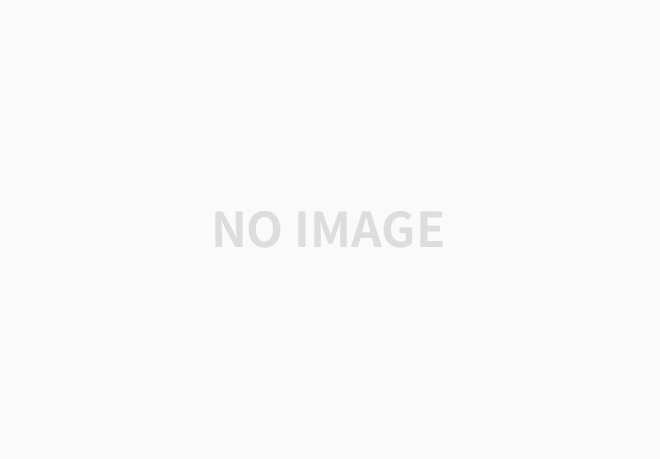
카트
1. 속성 - 이름
2. 행위 - 간다, 멈춘다, 드래프트
- 차
1. 속성 - 종류
2. 행위 - 묵직한 드래프트
- 캐릭터
1. 속성 - 종류
2. 행위 - 얆은 드리프트
- 기타
1. 속성 - 종류
2. 행위 - 떠서 간다
추상화된 cart 생성
package game;
public class cart {
protected String cName;
public cart(String cName) {
this.cName = cName;
}
public void start() {
System.out.println(this.cName + " 출발");
}
public void stop() {
System.out.println(this.cName + " 멈춤");
}
public void draft() {
System.out.println(this.cName + " 드래프트");
}
}
- 차 class
package game;
public class car extends cart{
private String kind;
public car(String cName, String kind) {
super(cName);
this.kind = kind;
}
public void draft() {
System.out.println(cName + " 묵직한 드래프트");
}
public String getKind() {
return this.kind;
}
}
- 캐릭터 class
package game;
public class charactor extends cart{
private String kind;
public charactor(String cName, String kind) {
super(cName);
this.kind = kind;
}
public void draft() {
System.out.println(cName + " 얆은 드리프트");
}
public String getKind() {
return this.kind;
}
}
- 기타 class
package game;
public class etc extends cart{
private String kind;
public etc(String cName, String kind) {
super(cName);
this.kind = kind;
}
public void start() {
System.out.println(cName + " 떠서 간다");
}
public String getKind() {
return this.kind;
}
}
- 만들어보기
package Pkg_car;
import game.*;
public class play {
public static void main(String[] args) {
car car1 = new car("파라곤", "스피드");
car1.start();
car1.stop();
car1.draft();
String car1Kind = car1.getKind();
System.out.println(car1Kind);
System.out.println("------------------------------");
charactor cha1 = new charactor("펭수", "아이템");
cha1.start();
cha1.stop();
cha1.draft();
String cha1Kind = cha1.getKind();
System.out.println(cha1Kind);
System.out.println("------------------------------");
etc e1 = new etc("칼", "스피드");
e1.start();
e1.stop();
e1.draft();
String e1Kind = e1.getKind();
System.out.println(e1Kind);
System.out.println("------------------------------");
}
}
'JAVA' 카테고리의 다른 글
[ JAVA ] Casting (upCasting/downCasting) - 객체타입끼리의 형변환 (0) | 2021.05.31 |
---|---|
[ JAVA ] 인터페이스(interface) - 특정 행위에 대한 추상화 (0) | 2021.05.28 |
[ JAVA ] 접근 제한자 - 어디까지 접근이 가능한가 (0) | 2021.05.25 |
[ JAVA ] 2차원 배열 - 배열의 배열 (0) | 2021.05.24 |
[ JAVA ] Call by Value vs Call by Reference (0) | 2021.05.20 |